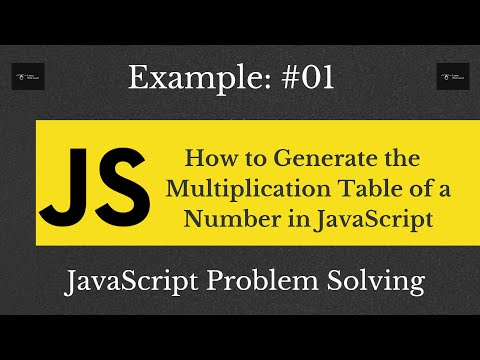
JavaScript is a versatile and powerful programming language widely used in web development. One of the common tasks developers face is converting length units from one measurement system to another. This task might involve switching between metric units like meters, centimeters, and millimeters or converting to and from imperial units such as inches, feet, and yards. fsiblog explores different techniques and best practices for converting length units in JavaScript.
Why Unit Conversion Matters
Unit conversion is essential in various applications, including:
Web Design and Development: Converting CSS units like pixels to percentages or ems.
Scientific Calculations: Handling different measurement systems for experiments.
E-commerce: Converting product dimensions for global customers.
Games: Adapting measurements for graphics or physics simulations.
Understanding how to handle conversions accurately can make your applications more versatile and user-friendly.
Understanding Length Units
Before diving into code, let’s briefly overview common length units:
Metric Units:
Millimeter (mm)
Centimeter (cm)
Meter (m)
Kilometer (km)
Imperial Units:
Inch (in)
Foot (ft)
Yard (yd)
Mile (mi)
CSS Units (used in web design):
Pixel (px)
Em
Percentage (%)
Viewport units (vw, vh)
Each of these units serves a specific purpose, and converting between them often involves multiplying by conversion factors.
Key Conversion Formulas
Here are some common conversion factors:
Metric Conversions:
1 meter = 100 centimeters
1 meter = 1000 millimeters
1 kilometer = 1000 meters
Imperial Conversions:
1 foot = 12 inches
1 yard = 3 feet
1 mile = 5280 feet
Metric to Imperial:
1 inch = 2.54 centimeters
1 meter = 39.3701 inches
1 kilometer = 0.621371 miles
Imperial to Metric:
1 centimeter = 0.393701 inches
1 foot = 30.48 centimeters
1 mile = 1.60934 kilometers
Writing a Unit Conversion Function in JavaScript
Let’s start by building a basic JavaScript function for length conversion. The function will handle conversions between various units by using the appropriate conversion factors.
function convertLength(value, fromUnit, toUnit) {
const conversionRates = {
// Metric to Metric
'mm': { 'cm': 0.1, 'm': 0.001, 'km': 0.000001 },
'cm': { 'mm': 10, 'm': 0.01, 'km': 0.00001 },
'm': { 'mm': 1000, 'cm': 100, 'km': 0.001 },
'km': { 'mm': 1000000, 'cm': 100000, 'm': 1000 },
// Imperial to Imperial
'in': { 'ft': 1 / 12, 'yd': 1 / 36, 'mi': 1 / 63360 },
'ft': { 'in': 12, 'yd': 1 / 3, 'mi': 1 / 5280 },
'yd': { 'in': 36, 'ft': 3, 'mi': 1 / 1760 },
'mi': { 'in': 63360, 'ft': 5280, 'yd': 1760 },
// Metric to Imperial
'mm': { 'in': 0.0393701 },
'cm': { 'in': 0.393701 },
'm': { 'in': 39.3701, 'ft': 3.28084, 'yd': 1.09361 },
'km': { 'mi': 0.621371 },
// Imperial to Metric
'in': { 'mm': 25.4, 'cm': 2.54, 'm': 0.0254 },
'ft': { 'm': 0.3048 },
'yd': { 'm': 0.9144 },
'mi': { 'km': 1.60934 }
};
if (!conversionRates[fromUnit] || !conversionRates[fromUnit][toUnit]) {
throw new Error(`Conversion from ${fromUnit} to ${toUnit} is not supported.`);
}
return value * conversionRates[fromUnit][toUnit];
}
// Example Usage
console.log(convertLength(100, 'cm', 'm')); // Output: 1
console.log(convertLength(1, 'km', 'mi')); // Output: 0.621371
How It Works
Conversion Rates: A nested object stores the conversion factors between units.
Input Validation: The function checks if the conversion is supported.
Calculation: It multiplies the input value by the corresponding conversion factor.
Output: Returns the converted value.
This function supports a wide range of conversions and can easily be extended by adding more units or conversion rates.
Converting CSS Units
CSS units like px, em, and % are relative and depend on the context, making their conversion more complex. For example:
Pixels (px): Absolute unit commonly used in web design.
Ems and Percentages: Relative units based on parent element sizes.
Viewport Units: Relative to the browser window size.
Let’s see how to convert between some of these units.
function convertPixelsToEms(pixels, baseFontSize = 16) {
return pixels / baseFontSize;
}
function convertEmsToPixels(ems, baseFontSize = 16) {
return ems * baseFontSize;
}
// Example Usage
console.log(convertPixelsToEms(32)); // Output: 2 (if base font size is 16px)
console.log(convertEmsToPixels(2)); // Output: 32 (if base font size is 16px)
Here, baseFontSize is usually 16 pixels, which is the default size for most browsers.
Handling User Input
When implementing unit conversion in a real-world application, user input must be validated to avoid errors. Here’s an example of a web-based length converter:
<input type="number" id="value" placeholder="Enter value">
<select id="fromUnit">
<option value="cm">Centimeter</option>
<option value="m">Meter</option>
<option value="in">Inch</option>
<option value="ft">Foot</option>
</select>
<select id="toUnit">
<option value="cm">Centimeter</option>
<option value="m">Meter</option>
<option value="in">Inch</option>
<option value="ft">Foot</option>
</select>
<button onclick="performConversion()">Convert</button>
<p id="result"></p>
<script>
function performConversion() {
const value = parseFloat(document.getElementById('value').value);
const fromUnit = document.getElementById('fromUnit').value;
const toUnit = document.getElementById('toUnit').value;
try {
const result = convertLength(value, fromUnit, toUnit);
document.getElementById('result').innerText = `${value} ${fromUnit} = ${result} ${toUnit}`;
} catch (error) {
document.getElementById('result').innerText = error.message;
}
}
</script>
This basic UI allows users to enter a value, select units, and see the conversion result. It leverages the convertLength function we wrote earlier.
Dealing with Edge Cases
Some edge cases to handle in unit conversion:
Invalid Input: Non-numeric or negative values.
Solution: Validate input before performing the conversion.
Unsupported Units: Converting between unrelated units.
Solution: Check if the conversion is supported and throw an error if not.
Precision: Floating-point arithmetic errors.
Solution: Use toFixed() or other rounding methods to manage precision.
Dynamic Context (CSS Units): Values like em and % depend on parent elements or viewport size.
Solution: Use JavaScript to dynamically fetch context-specific values.
Advanced Techniques: Using Libraries
For complex conversions, consider using libraries like convert-units:
npm install convert-units
const convert = require('convert-units');
// Example Usage
console.log(convert(1).from('m').to('cm')); // Output: 100
console.log(convert(10).from('in').to('cm')); // Output: 25.4
This library supports a wide range of units and simplifies the process of managing conversion factors.
Conclusion
Converting length units in JavaScript is a crucial skill for developers across many domains. Whether you’re building scientific calculators, responsive web designs, or global e-commerce platforms, understanding and implementing accurate unit conversions is essential. By following the techniques outlined in this article and leveraging tools like libraries for advanced scenarios, you can handle any unit conversion task with confidence.